Computer Instructions
The basic computer utilizes three distinct instruction code formats, each consisting of 16 bits. The operation code (opcode) part of the instruction contains three bits, and the meaning of the remaining 13 bits varies depending on the opcode.
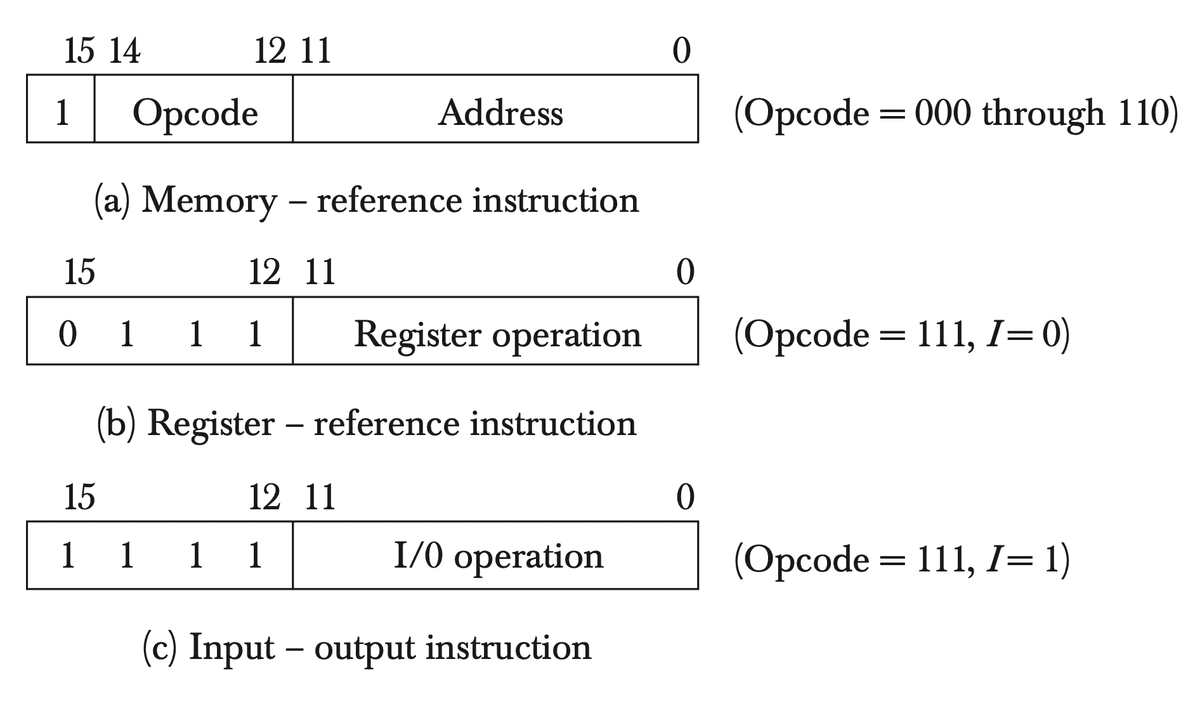
Instruction Formats
Memory-Reference Instructions
A memory-reference instruction uses 12 bits to specify an address and one bit to specify the addressing mode (denoted as ( I )). The addressing mode bit (( I )) is equal to 0 for direct addressing and 1 for indirect addressing. This format is structured as follows:
- Bits 15-12: Opcode (000 through 110)
- Bit 15: Addressing mode ( I ) (0 for direct, 1 for indirect)
- Bits 11-0: Address
Register-Reference Instructions
Register-reference instructions are identified by the opcode 111 with a 0 in the leftmost bit (bit 15). These instructions specify operations or tests on the AC (Accumulator) register and do not require an operand from memory. The format is:
- Bits 15-12: 111 (Opcode)
- Bit 15: 0 (Register operation indicator)
- Bits 11-0: Operation or test
Input-Output Instructions
Input-output instructions use the opcode 111 with a 1 in the leftmost bit (bit 15). These instructions specify the type of input-output operation or test. The format is:
- Bits 15-12: 111 (Opcode)
- Bit 15: 1 (I/O operation indicator)
- Bits 11-0: Type of I/O operation or test
Instruction Type Recognition
The type of instruction is determined by the computer control unit by examining the four bits in positions 12 through 15 of the instruction. The process is as follows:
-
Memory-Reference Instruction:
- If the opcode bits (12-14) are not equal to 111, the instruction is a memory-reference type.
- The bit in position 15 is then interpreted as the addressing mode ( I ).
-
Register-Reference Instruction:
- If the opcode bits (12-14) equal 111 and the bit in position 15 is 0, the instruction is a register-reference type.
-
Input-Output Instruction:
- If the opcode bits (12-14) equal 111 and the bit in position 15 is 1, the instruction is an input-output type.
Note that the bit in position 15 is designated as ( I ) but is not used as a mode bit when the opcode equals 111.
Instruction Set and Hexadecimal Representation
Although only three bits are used for the opcode, the instruction set is not limited to eight operations. Register-reference and input-output instructions utilize the remaining 12 bits as part of their operation code, allowing for a total of 25 distinct instructions in the basic computer.
The instructions are listed in the table below:
Hexadecimal Code | Symbol | Description |
---|---|---|
0xxx | AND | AND memory word to AC |
1xxx | ADD | Add memory word to AC |
2xxx | LDA | Load memory word to AC |
3xxx | STA | Store content of AC in memory |
4xxx | BUN | Branch unconditionally |
5xxx | BSA | Branch and save return address |
6xxx | ISZ | Increment and skip if zero |
7800 | CLA | Clear AC |
7400 | CLE | Clear E |
7200 | CMA | Complement AC |
7100 | CME | Complement E |
7080 | CIR | Circulate right AC and E |
7040 | CIL | Circulate left AC and E |
7020 | INC | Increment AC |
7010 | SPA | Skip next instruction if AC positive |
7008 | SNA | Skip next instruction if AC negative |
7004 | SZA | Skip next instruction if AC zero |
7002 | SZE | Skip next instruction if E is 0 |
7001 | HLT | Halt computer |
F800 | INP | Input character to AC |
F400 | OUT | Output character from AC |
F200 | SKI | Skip on input flag |
F100 | SKO | Skip on output flag |
F080 | ION | Interrupt on |
F040 | IOF | Interrupt off |
The symbol designation is a three-letter abbreviation for the instruction's function. The hexadecimal code reduces the 16 bits of the instruction code to four digits, each representing four bits.
Addressing and Hexadecimal Codes
- Memory-reference instructions have a 12-bit address part, denoted by three x's (xxx), corresponding to the three hexadecimal digits.
- The last bit of the instruction is designated by ( I ). When ( I = 0 ), the last four bits range from 0 to 6 (hexadecimal). When ( I = 1 ), the range is 8 to E (hexadecimal).
Register-Reference Instructions
- Use all 16 bits to specify an operation.
- The leftmost four bits are always 0111 (hexadecimal 7), with the remaining bits specifying the operation.
Input-Output Instructions
- Also use all 16 bits.
- The last four bits are always 1111 (hexadecimal F), with the remaining bits specifying the operation.
Instruction Set Completeness
To ensure that the computer can execute any computable function, it must include a complete set of instructions covering the following categories:
- Arithmetic, logical, and shift instructions.
- Instructions for moving information between memory and processor registers.
- Program control instructions and status condition checks.
- Input and output instructions.
Detailed Instruction Functions
-
Arithmetic and Logical Instructions:
- ADD: Add memory word to AC.
- CMA: Complement AC.
- INC: Increment AC.
- AND: Perform logical AND on memory word and AC.
- CLA: Clear AC.
- CLE: Clear E.
-
Shift Instructions:
- CIR: Circulate right AC and E.
- CIL: Circulate left AC and E.
-
Control and Status Instructions:
- BUN: Unconditional branch.
- BSA: Branch and save return address.
- ISZ: Increment and skip if zero.
- SPA: Skip next instruction if AC positive.
- SNA: Skip next instruction if AC negative.
- SZA: Skip next instruction if AC zero.
- SZE: Skip next instruction if E is zero.
- HLT: Halt the computer.
-
Input and Output Instructions:
- INP: Input character to AC.
- OUT: Output character from AC.
- SKI: Skip on input flag.
- SKO: Skip on output flag.
- ION: Interrupt on.
- IOF: Interrupt off.
The basic computer's instruction set, while minimal, is complete and capable of performing necessary operations for general-purpose computation. Despite its simplicity, it demonstrates fundamental principles of computer organization and design. More efficient and complex instruction sets are found in commercial computers, but the basic set provides a clear foundation for understanding computer architecture.